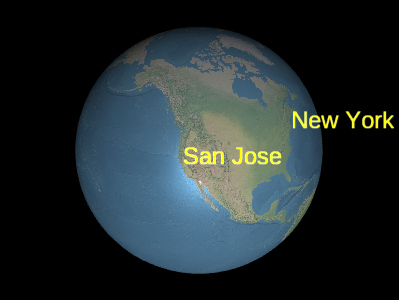
示例标签
使用
LabelCollection#add
和
LabelCollection#remove
从集合中添加和删除标签。
Performance:
为了获得最佳性能,最好选择几个集合,每个集合都有很多标签,而不是多个集合,每个集合只有几个标签。避免有一些标签每帧都会改变而其他标签不会改变的集合;相反,为静态标签创建一个或多个集合,为动态标签创建一个或多个集合。
Name | Type | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options
|
Object |
具有以下属性的
可选
对象:
|
Example:
// Create a label collection with two labels
const labels = scene.primitives.add(new Cesium.LabelCollection());
labels.add({
position : new Cesium.Cartesian3(1.0, 2.0, 3.0),
text : 'A label'
});
labels.add({
position : new Cesium.Cartesian3(4.0, 5.0, 6.0),
text : 'Another label'
});
Demo:
See:
Members
blendOption : BlendOption
-
Default Value:
BlendOption.OPAQUE_AND_TRANSLUCENT
为图元中的每个绘制命令绘制边界球体。
-
Default Value:
false
LabelCollection#get
一起使用以遍历集合中的所有标签。
modelMatrix : Matrix4
Transforms.eastNorthUpToFixedFrame
返回的那样。
-
Default Value:
Matrix4.IDENTITY
Example:
const center = Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883);
labels.modelMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center);
labels.add({
position : new Cesium.Cartesian3(0.0, 0.0, 0.0),
text : 'Center'
});
labels.add({
position : new Cesium.Cartesian3(1000000.0, 0.0, 0.0),
text : 'East'
});
labels.add({
position : new Cesium.Cartesian3(0.0, 1000000.0, 0.0),
text : 'North'
});
labels.add({
position : new Cesium.Cartesian3(0.0, 0.0, 1000000.0),
text : 'Up'
});
-
Default Value:
true
Methods
add ( options ) → Label
Performance:
调用
add
是预期的恒定时间。但是,集合的顶点缓冲区被重写;此操作是
O(n)
并且还会导致 CPU 到 GPU 开销。为了获得最佳性能,请在调用
update
之前添加尽可能多的广告牌。
Name | Type | Description |
---|---|---|
options
|
Object | 可选 描述标签属性的模板,如示例 1 所示。 |
Returns:
Throws:
-
DeveloperError : 该对象被销毁,即调用了destroy()。
Examples:
// Example 1: Add a label, specifying all the default values.
const l = labels.add({
show : true,
position : Cesium.Cartesian3.ZERO,
text : '',
font : '30px sans-serif',
fillColor : Cesium.Color.WHITE,
outlineColor : Cesium.Color.BLACK,
outlineWidth : 1.0,
showBackground : false,
backgroundColor : new Cesium.Color(0.165, 0.165, 0.165, 0.8),
backgroundPadding : new Cesium.Cartesian2(7, 5),
style : Cesium.LabelStyle.FILL,
pixelOffset : Cesium.Cartesian2.ZERO,
eyeOffset : Cesium.Cartesian3.ZERO,
horizontalOrigin : Cesium.HorizontalOrigin.LEFT,
verticalOrigin : Cesium.VerticalOrigin.BASELINE,
scale : 1.0,
translucencyByDistance : undefined,
pixelOffsetScaleByDistance : undefined,
heightReference : HeightReference.NONE,
distanceDisplayCondition : undefined
});
// Example 2: Specify only the label's cartographic position,
// text, and font.
const l = labels.add({
position : Cesium.Cartesian3.fromRadians(longitude, latitude, height),
text : 'Hello World',
font : '24px Helvetica',
});
See:
Name | Type | Description |
---|---|---|
label
|
Label | 要检查的标签。 |
Returns:
See:
一旦一个对象被销毁,它就不应该被使用;调用
isDestroyed
以外的任何函数都将导致
DeveloperError
异常。因此,如示例中所做的那样,将返回值 (
undefined
) 分配给对象。
Throws:
-
DeveloperError : 该对象被销毁,即调用了destroy()。
Example:
labels = labels && labels.destroy();
See:
get (index) → Label
LabelCollection#length
一起使用,以遍历集合中的所有标签。
Performance:
预期的恒定时间。如果从集合中删除标签并且未调用
Scene#render
,则执行隐式
O(n)
操作。
Name | Type | Description |
---|---|---|
index
|
Number | 广告牌的从零开始的索引。 |
Returns:
Throws:
-
DeveloperError : 该对象被销毁,即调用了destroy()。
Example:
// Toggle the show property of every label in the collection
const len = labels.length;
for (let i = 0; i < len; ++i) {
const l = billboards.get(i);
l.show = !l.show;
}
See:
Returns:
Performance:
调用
remove
是预期的恒定时间。但是,集合的顶点缓冲区被重写 - 一个
O(n)
操作,也会导致 CPU 到 GPU 开销。为了获得最佳性能,请在调用
update
之前删除尽可能多的标签。如果您打算暂时隐藏标签,调用
Label#show
通常比删除并重新添加标签更有效。
Name | Type | Description |
---|---|---|
label
|
Label | 要删除的标签。 |
Returns:
true
;如果在集合中找不到标签,则
false
。
Throws:
-
DeveloperError : 该对象被销毁,即调用了destroy()。
Example:
const l = labels.add(...);
labels.remove(l); // Returns true
See:
Performance:
O(n)
。从集合中删除所有标签然后添加新标签比完全创建新集合更有效。
Throws:
-
DeveloperError : 该对象被销毁,即调用了destroy()。
Example:
labels.add(...);
labels.add(...);
labels.removeAll();