Performance:
读取一个属性,例如
Billboard#show
,是一个常数时间。分配给属性是恒定的时间,但在调用
BillboardCollection#update
时会导致 CPU 到 GPU 的流量。无论更新了多少属性,每个广告牌的流量都是相同的。如果集合中的大多数广告牌需要更新,使用
BillboardCollection#removeAll
清除集合并添加新广告牌而不是修改每个广告牌可能会更有效。
Throws:
-
DeveloperError : scaleByDistance.far 必须大于 scaleByDistance.near
-
DeveloperError : translucencyByDistance.far 必须大于 translucencyByDistance.near
-
DeveloperError : pixelOffsetScaleByDistance.far 必须大于 pixelOffsetScaleByDistance.near
-
DeveloperError : distanceDisplayCondition.far 必须大于 distanceDisplayCondition.near
Members
alignedAxis : Cartesian3
Examples:
// Example 1.
// Have the billboard up vector point north
billboard.alignedAxis = Cesium.Cartesian3.UNIT_Z;
// Example 2.
// Have the billboard point east.
billboard.alignedAxis = Cesium.Cartesian3.UNIT_Z;
billboard.rotation = -Cesium.Math.PI_OVER_TWO;
// Example 3.
// Reset the aligned axis
billboard.alignedAxis = Cesium.Cartesian3.ZERO;
color : Color
0.0
使广告牌透明,而
1.0
使广告牌不透明。
default
![]() |
alpha : 0.5
![]() |
红色、绿色、蓝色和 alpha 值由
value
的
red
、
green
、
blue
和
alpha
属性表示,如示例 1 所示。这些分量的范围从
0.0
(无强度)到
1.0
(全强度)。
Examples:
// Example 1. Assign yellow.
b.color = Cesium.Color.YELLOW;
// Example 2. Make a billboard 50% translucent.
b.color = new Cesium.Color(1.0, 1.0, 1.0, 0.5);
distanceDisplayCondition : DistanceDisplayCondition
-
Default Value:
undefined
eyeOffset : Cartesian3
x
指向观看者的右侧,
y
指向上方,
z
指向屏幕内。眼睛坐标使用与世界和模型坐标相同的比例,通常为米。
眼睛偏移通常用于将多个广告牌或对象布置在同一位置,例如,将广告牌布置在其对应的 3D 模型上方。
下面,广告牌位于地球的中心,但视线偏移使其始终出现在地球顶部,无论观看者或地球的方向如何。
![]() |
![]() |
b.eyeOffset = new Cartesian3(0.0, 8000000.0, 0.0);
heightReference : HeightReference
-
Default Value:
HeightReference.NONE
horizontalOrigin : HorizontalOrigin
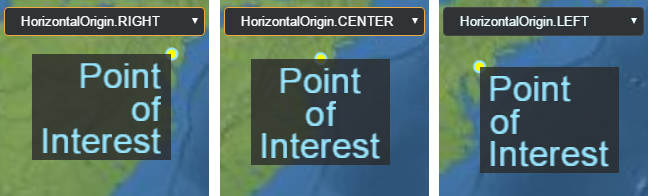
Example:
// Use a bottom, left origin
b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT;
b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
获取或设置用于此广告牌的图像。如果已经为给定图像创建了纹理,则使用现有纹理。
此属性可以设置为加载的图像、将自动加载为图像的 URL、画布或另一个广告牌的图像属性(来自同一个广告牌集合)。
Example:
// load an image from a URL
b.image = 'some/image/url.png';
// assuming b1 and b2 are billboards in the same billboard collection,
// use the same image for both billboards.
b2.image = b1.image;
pixelOffset : Cartesian2
x
从左到右增加,
y
从上到下增加。
default
![]() |
b.pixeloffset = new Cartesian2(50, 25);
![]() |
pixelOffsetScaleByDistance : NearFarScalar
NearFarScalar#nearValue
和
NearFarScalar#farValue
之间缩放,而相机距离落在指定的
NearFarScalar#near
和
NearFarScalar#far
的上下限内。在这些范围之外,广告牌的像素偏移比例保持在最近的范围内。如果未定义,pixelOffsetScaleByDistance 将被禁用。
Examples:
// Example 1.
// Set a billboard's pixel offset scale to 0.0 when the
// camera is 1500 meters from the billboard and scale pixel offset to 10.0 pixels
// in the y direction the camera distance approaches 8.0e6 meters.
b.pixelOffset = new Cesium.Cartesian2(0.0, 1.0);
b.pixelOffsetScaleByDistance = new Cesium.NearFarScalar(1.5e2, 0.0, 8.0e6, 10.0);
// Example 2.
// disable pixel offset by distance
b.pixelOffsetScaleByDistance = undefined;
position : Cartesian3
true
,则该广告牌已准备好渲染,即图像已下载并创建了 WebGL 资源。
-
Default Value:
false
1.0
的比例不会改变广告牌的大小;大于
1.0
的比例会放大广告牌;小于
1.0
的正比例会缩小广告牌。
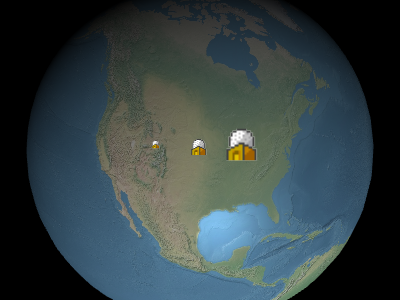
上图中从左到右,比例分别为
0.5
、
1.0
和
2.0
。
scaleByDistance : NearFarScalar
NearFarScalar#near
和
NearFarScalar#far
的上下限内时,广告牌的比例将在
NearFarScalar#nearValue
和
NearFarScalar#farValue
之间进行插值。在这些范围之外,广告牌的比例保持在最近的范围内。如果未定义,scaleByDistance 将被禁用。
Examples:
// Example 1.
// Set a billboard's scaleByDistance to scale by 1.5 when the
// camera is 1500 meters from the billboard and disappear as
// the camera distance approaches 8.0e6 meters.
b.scaleByDistance = new Cesium.NearFarScalar(1.5e2, 1.5, 8.0e6, 0.0);
// Example 2.
// disable scaling by distance
b.scaleByDistance = undefined;
-
Default Value:
true
true
的以米为单位的广告牌大小;否则,大小以像素为单位。
-
Default Value:
false
translucencyByDistance : NearFarScalar
NearFarScalar#nearValue
和
NearFarScalar#farValue
之间进行插值,而相机距离落在指定的
NearFarScalar#near
和
NearFarScalar#far
的上下限内。在这些范围之外,广告牌的半透明度仍然被限制在最近的范围内。如果未定义,则 translucencyByDistance 将被禁用。
Examples:
// Example 1.
// Set a billboard's translucency to 1.0 when the
// camera is 1500 meters from the billboard and disappear as
// the camera distance approaches 8.0e6 meters.
b.translucencyByDistance = new Cesium.NearFarScalar(1.5e2, 1.0, 8.0e6, 0.0);
// Example 2.
// disable translucency by distance
b.translucencyByDistance = undefined;
verticalOrigin : VerticalOrigin
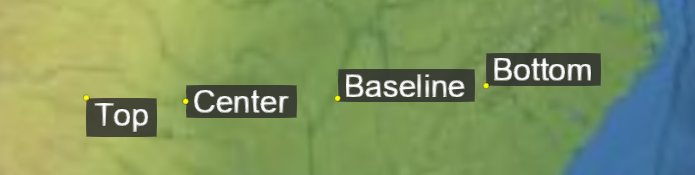
Example:
// Use a bottom, left origin
b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT;
b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
Methods
computeScreenSpacePosition (scene, result ) → Cartesian2
x
从左到右增加,
y
从上到下增加。
Name | Type | Description |
---|---|---|
scene
|
Scene | 现场。 |
result
|
Cartesian2 | 可选 存储结果的对象。 |
Returns:
Throws:
-
DeveloperError : 广告牌必须在一个集合中。
Example:
console.log(b.computeScreenSpacePosition(scene).toString());
See:
Name | Type | Description |
---|---|---|
other
|
Billboard | 用于比较平等的广告牌。 |
Returns:
true
;否则,
false
。
设置要用于此广告牌的图像。如果已经为给定的 id 创建了纹理,则使用现有的纹理。
此功能对于动态创建在许多广告牌之间共享的纹理很有用。只有第一个广告牌会实际调用该函数并创建纹理,而使用相同 id 创建的后续广告牌将简单地重新使用现有纹理。
要从 URL 加载图像,设置
Billboard#image
属性更方便。
Name | Type | Description |
---|---|---|
id
|
String | 图片的id。这可以是唯一标识图像的任何字符串。 |
image
|
HTMLImageElement | HTMLCanvasElement | String | Resource | Billboard.CreateImageCallback | 要加载的图像。该参数可以是加载的 Image 或 Canvas,一个将自动加载为 Image 的 URL,也可以是一个函数,如果尚未加载则将调用该函数来创建该图像。 |
Example:
// create a billboard image dynamically
function drawImage(id) {
// create and draw an image using a canvas
const canvas = document.createElement('canvas');
const context2D = canvas.getContext('2d');
// ... draw image
return canvas;
}
// drawImage will be called to create the texture
b.setImage('myImage', drawImage);
// subsequent billboards created in the same collection using the same id will use the existing
// texture, without the need to create the canvas or draw the image
b2.setImage('myImage', drawImage);
Name | Type | Description |
---|---|---|
id
|
String | 要使用的图像的 ID。 |
subRegion
|
BoundingRectangle | 图像的子区域。 |
Throws:
-
RuntimeError : 带有 id 的图像必须在图集中
Type Definitions
Cesium.Billboard.CreateImageCallback (id) → HTMLImageElement|HTMLCanvasElement|Promise.<(HTMLImageElement|HTMLCanvasElement)>
Name | Type | Description |
---|---|---|
id
|
String | 要加载的图像的标识符。 |