Name | Type | Description |
---|---|---|
options |
LabelGraphics.ConstructorOptions | optional Object describing initialization options |
Members
backgroundColor : Property|undefined
Gets or sets the Property specifying the background
Color
.
-
Default Value:
new Color(0.165, 0.165, 0.165, 0.8)
backgroundPadding : Property|undefined
Gets or sets the
Cartesian2
Property specifying the label's horizontal and vertical
background padding in pixels.
-
Default Value:
new Cartesian2(7, 5)
readonly definitionChanged : Event
Gets the event that is raised whenever a property or sub-property is changed or modified.
disableDepthTestDistance : Property|undefined
Gets or sets the distance from the camera at which to disable the depth test to, for example, prevent clipping against terrain.
When set to zero, the depth test is always applied. When set to Number.POSITIVE_INFINITY, the depth test is never applied.
distanceDisplayCondition : Property|undefined
Gets or sets the
DistanceDisplayCondition
Property specifying at what distance from the camera that this label will be displayed.
eyeOffset : Property|undefined
Gets or sets the
Cartesian3
Property specifying the label's offset in eye coordinates.
Eye coordinates is a left-handed coordinate system, where x
points towards the viewer's
right, y
points up, and z
points into the screen.
An eye offset is commonly used to arrange multiple labels or objects at the same position, e.g., to arrange a label above its corresponding 3D model.
Below, the label is positioned at the center of the Earth but an eye offset makes it always appear on top of the Earth regardless of the viewer's or Earth's orientation.
![]() |
![]() |
l.eyeOffset = new Cartesian3(0.0, 8000000.0, 0.0);
-
Default Value:
Cartesian3.ZERO
fillColor : Property|undefined
Gets or sets the Property specifying the fill
Color
.
font : Property|undefined
Gets or sets the string Property specifying the font in CSS syntax.
See:
heightReference : Property|undefined
Gets or sets the Property specifying the
HeightReference
.
-
Default Value:
HeightReference.NONE
horizontalOrigin : Property|undefined
Gets or sets the Property specifying the
HorizontalOrigin
.
outlineColor : Property|undefined
Gets or sets the Property specifying the outline
Color
.
outlineWidth : Property|undefined
Gets or sets the numeric Property specifying the outline width.
pixelOffset : Property|undefined
Gets or sets the
The label's origin is indicated by the yellow point.
Cartesian2
Property specifying the label's pixel offset in screen space
from the origin of this label. This is commonly used to align multiple labels and labels at
the same position, e.g., an image and text. The screen space origin is the top, left corner of the
canvas; x
increases from left to right, and y
increases from top to bottom.
default ![]() |
l.pixeloffset = new Cartesian2(25, 75); ![]() |
-
Default Value:
Cartesian2.ZERO
pixelOffsetScaleByDistance : Property|undefined
Gets or sets
NearFarScalar
Property specifying the pixel offset of the label based on the distance from the camera.
A label's pixel offset will interpolate between the NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the label's pixel offset remains clamped to the nearest bound.
scale : Property|undefined
Gets or sets the numeric Property specifying the uniform scale to apply to the image.
A scale greater than
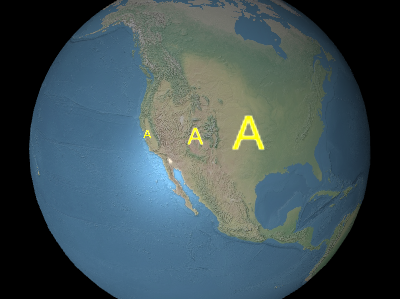
From left to right in the above image, the scales are
1.0
enlarges the label while a scale less than 1.0
shrinks it.
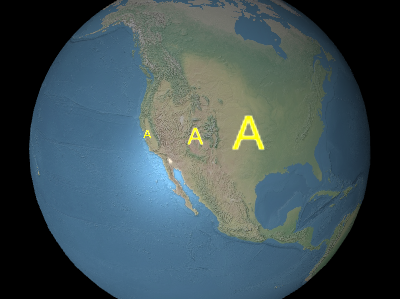
From left to right in the above image, the scales are
0.5
, 1.0
,
and 2.0
.
-
Default Value:
1.0
scaleByDistance : Property|undefined
Gets or sets near and far scaling properties of a Label based on the label's distance from the camera.
A label's scale will interpolate between the
NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the label's scale remains clamped to the nearest bound. If undefined,
scaleByDistance will be disabled.
show : Property|undefined
Gets or sets the boolean Property specifying the visibility of the label.
showBackground : Property|undefined
Gets or sets the boolean Property specifying the visibility of the background behind the label.
-
Default Value:
false
style : Property|undefined
Gets or sets the Property specifying the
LabelStyle
.
text : Property|undefined
Gets or sets the string Property specifying the text of the label.
Explicit newlines '\n' are supported.
translucencyByDistance : Property|undefined
Gets or sets
NearFarScalar
Property specifying the translucency of the label based on the distance from the camera.
A label's translucency will interpolate between the NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the label's translucency remains clamped to the nearest bound.
verticalOrigin : Property|undefined
Gets or sets the Property specifying the
VerticalOrigin
.
Methods
clone(result) → LabelGraphics
Duplicates this instance.
Name | Type | Description |
---|---|---|
result |
LabelGraphics | optional The object onto which to store the result. |
Returns:
The modified result parameter or a new instance if one was not provided.
Assigns each unassigned property on this object to the value
of the same property on the provided source object.
Name | Type | Description |
---|---|---|
source |
LabelGraphics | The object to be merged into this object. |
Type Definitions
Initialization options for the LabelGraphics constructor
Properties:
Name | Type | Attributes | Default | Description |
---|---|---|---|---|
show |
Property | boolean |
<optional> |
true | A boolean Property specifying the visibility of the label. |
text |
Property | string |
<optional> |
A Property specifying the text. Explicit newlines '\n' are supported. | |
font |
Property | string |
<optional> |
'30px sans-serif' | A Property specifying the CSS font. |
style |
Property | LabelStyle |
<optional> |
LabelStyle.FILL | A Property specifying the LabelStyle . |
scale |
Property | number |
<optional> |
1.0 | A numeric Property specifying the scale to apply to the text. |
showBackground |
Property | boolean |
<optional> |
false | A boolean Property specifying the visibility of the background behind the label. |
backgroundColor |
Property | Color |
<optional> |
new Color(0.165, 0.165, 0.165, 0.8) | A Property specifying the background Color . |
backgroundPadding |
Property | Cartesian2 |
<optional> |
new Cartesian2(7, 5) | A Cartesian2 Property specifying the horizontal and vertical background padding in pixels. |
pixelOffset |
Property | Cartesian2 |
<optional> |
Cartesian2.ZERO | A Cartesian2 Property specifying the pixel offset. |
eyeOffset |
Property | Cartesian3 |
<optional> |
Cartesian3.ZERO | A Cartesian3 Property specifying the eye offset. |
horizontalOrigin |
Property | HorizontalOrigin |
<optional> |
HorizontalOrigin.CENTER | A Property specifying the HorizontalOrigin . |
verticalOrigin |
Property | VerticalOrigin |
<optional> |
VerticalOrigin.CENTER | A Property specifying the VerticalOrigin . |
heightReference |
Property | HeightReference |
<optional> |
HeightReference.NONE | A Property specifying what the height is relative to. |
fillColor |
Property | Color |
<optional> |
Color.WHITE | A Property specifying the fill Color . |
outlineColor |
Property | Color |
<optional> |
Color.BLACK | A Property specifying the outline Color . |
outlineWidth |
Property | number |
<optional> |
1.0 | A numeric Property specifying the outline width. |
translucencyByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to set translucency based on distance from the camera. |
|
pixelOffsetScaleByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to set pixelOffset based on distance from the camera. |
|
scaleByDistance |
Property | NearFarScalar |
<optional> |
A NearFarScalar Property used to set scale based on distance from the camera. |
|
distanceDisplayCondition |
Property | DistanceDisplayCondition |
<optional> |
A Property specifying at what distance from the camera that this label will be displayed. | |
disableDepthTestDistance |
Property | number |
<optional> |
A Property specifying the distance from the camera at which to disable the depth test to. |