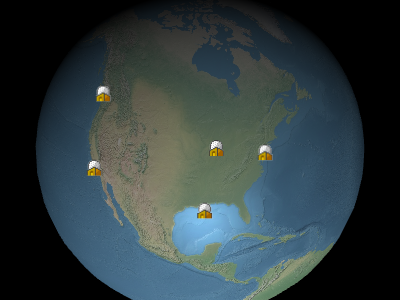
Example billboards
Billboards are added and removed from the collection using
BillboardCollection#add
and BillboardCollection#remove
. Billboards in a collection automatically share textures
for images with the same identifier.
Performance:
For best performance, prefer a few collections, each with many billboards, to many collections with only a few billboards each. Organize collections so that billboards with the same update frequency are in the same collection, i.e., billboards that do not change should be in one collection; billboards that change every frame should be in another collection; and so on.
Name | Type | Description | ||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
options |
Object |
optional
Object with the following properties:
|
Example:
// Create a billboard collection with two billboards
const billboards = scene.primitives.add(new Cesium.BillboardCollection());
billboards.add({
position : new Cesium.Cartesian3(1.0, 2.0, 3.0),
image : 'url/to/image'
});
billboards.add({
position : new Cesium.Cartesian3(4.0, 5.0, 6.0),
image : 'url/to/another/image'
});
Demo:
See:
Members
blendOption : BlendOption
-
Default Value:
BlendOption.OPAQUE_AND_TRANSLUCENT
Draws the bounding sphere for each draw command in the primitive.
-
Default Value:
false
Draws the texture atlas for this BillboardCollection as a fullscreen quad.
-
Default Value:
false
BillboardCollection#get
to iterate over all the billboards
in the collection.
modelMatrix : Matrix4
Transforms.eastNorthUpToFixedFrame
.
-
Default Value:
Matrix4.IDENTITY
Example:
const center = Cesium.Cartesian3.fromDegrees(-75.59777, 40.03883);
billboards.modelMatrix = Cesium.Transforms.eastNorthUpToFixedFrame(center);
billboards.add({
image : 'url/to/image',
position : new Cesium.Cartesian3(0.0, 0.0, 0.0) // center
});
billboards.add({
image : 'url/to/image',
position : new Cesium.Cartesian3(1000000.0, 0.0, 0.0) // east
});
billboards.add({
image : 'url/to/image',
position : new Cesium.Cartesian3(0.0, 1000000.0, 0.0) // north
});
billboards.add({
image : 'url/to/image',
position : new Cesium.Cartesian3(0.0, 0.0, 1000000.0) // up
});
See:
-
Default Value:
true
Methods
add(options) → Billboard
Performance:
Calling add
is expected constant time. However, the collection's vertex buffer
is rewritten - an O(n)
operation that also incurs CPU to GPU overhead. For
best performance, add as many billboards as possible before calling update
.
Name | Type | Description |
---|---|---|
options |
Object | optional A template describing the billboard's properties as shown in Example 1. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Examples:
// Example 1: Add a billboard, specifying all the default values.
const b = billboards.add({
show : true,
position : Cesium.Cartesian3.ZERO,
pixelOffset : Cesium.Cartesian2.ZERO,
eyeOffset : Cesium.Cartesian3.ZERO,
heightReference : Cesium.HeightReference.NONE,
horizontalOrigin : Cesium.HorizontalOrigin.CENTER,
verticalOrigin : Cesium.VerticalOrigin.CENTER,
scale : 1.0,
image : 'url/to/image',
imageSubRegion : undefined,
color : Cesium.Color.WHITE,
id : undefined,
rotation : 0.0,
alignedAxis : Cesium.Cartesian3.ZERO,
width : undefined,
height : undefined,
scaleByDistance : undefined,
translucencyByDistance : undefined,
pixelOffsetScaleByDistance : undefined,
sizeInMeters : false,
distanceDisplayCondition : undefined
});
// Example 2: Specify only the billboard's cartographic position.
const b = billboards.add({
position : Cesium.Cartesian3.fromDegrees(longitude, latitude, height)
});
See:
Name | Type | Description |
---|---|---|
billboard |
Billboard | optional The billboard to check for. |
Returns:
Once an object is destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception. Therefore,
assign the return value (undefined
) to the object as done in the example.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
billboards = billboards && billboards.destroy();
See:
get(index) → Billboard
BillboardCollection#length
to iterate over all the billboards
in the collection.
Performance:
Expected constant time. If billboards were removed from the collection and
BillboardCollection#update
was not called, an implicit O(n)
operation is performed.
Name | Type | Description |
---|---|---|
index |
Number | The zero-based index of the billboard. |
Returns:
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
// Toggle the show property of every billboard in the collection
const len = billboards.length;
for (let i = 0; i < len; ++i) {
const b = billboards.get(i);
b.show = !b.show;
}
See:
If this object was destroyed, it should not be used; calling any function other than
isDestroyed
will result in a DeveloperError
exception.
Returns:
true
if this object was destroyed; otherwise, false
.
Performance:
Calling remove
is expected constant time. However, the collection's vertex buffer
is rewritten - an O(n)
operation that also incurs CPU to GPU overhead. For
best performance, remove as many billboards as possible before calling update
.
If you intend to temporarily hide a billboard, it is usually more efficient to call
Billboard#show
instead of removing and re-adding the billboard.
Name | Type | Description |
---|---|---|
billboard |
Billboard | The billboard to remove. |
Returns:
true
if the billboard was removed; false
if the billboard was not found in the collection.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
const b = billboards.add(...);
billboards.remove(b); // Returns true
See:
Performance:
O(n)
. It is more efficient to remove all the billboards
from a collection and then add new ones than to create a new collection entirely.
Throws:
-
DeveloperError : This object was destroyed, i.e., destroy() was called.
Example:
billboards.add(...);
billboards.add(...);
billboards.removeAll();
See:
Viewer
or CesiumWidget
render the scene to
get the draw commands needed to render this primitive.
Do not call this function directly. This is documented just to list the exceptions that may be propagated when the scene is rendered:
Throws:
-
RuntimeError : image with id must be in the atlas.