BillboardCollection
. A billboard is created and its initial
properties are set by calling BillboardCollection#add
.
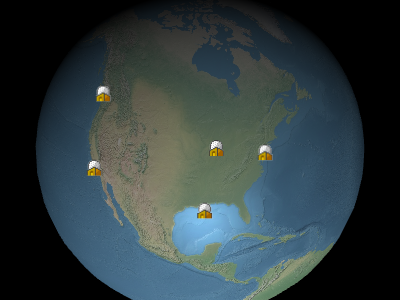
Example billboards
Performance:
Reading a property, e.g., Billboard#show
, is constant time.
Assigning to a property is constant time but results in
CPU to GPU traffic when BillboardCollection#update
is called. The per-billboard traffic is
the same regardless of how many properties were updated. If most billboards in a collection need to be
updated, it may be more efficient to clear the collection with BillboardCollection#removeAll
and add new billboards instead of modifying each one.
Throws:
-
DeveloperError : scaleByDistance.far must be greater than scaleByDistance.near
-
DeveloperError : translucencyByDistance.far must be greater than translucencyByDistance.near
-
DeveloperError : pixelOffsetScaleByDistance.far must be greater than pixelOffsetScaleByDistance.near
-
DeveloperError : distanceDisplayCondition.far must be greater than distanceDisplayCondition.near
Members
alignedAxis : Cartesian3
Examples:
// Example 1.
// Have the billboard up vector point north
billboard.alignedAxis = Cesium.Cartesian3.UNIT_Z;
// Example 2.
// Have the billboard point east.
billboard.alignedAxis = Cesium.Cartesian3.UNIT_Z;
billboard.rotation = -Cesium.Math.PI_OVER_TWO;
// Example 3.
// Reset the aligned axis
billboard.alignedAxis = Cesium.Cartesian3.ZERO;
color : Color
0.0
makes the billboard transparent, and 1.0
makes the billboard opaque.
default ![]() |
alpha : 0.5 ![]() |
The red, green, blue, and alpha values are indicated by
value
's red
, green
,
blue
, and alpha
properties as shown in Example 1. These components range from 0.0
(no intensity) to 1.0
(full intensity).
Examples:
// Example 1. Assign yellow.
b.color = Cesium.Color.YELLOW;
// Example 2. Make a billboard 50% translucent.
b.color = new Cesium.Color(1.0, 1.0, 1.0, 0.5);
distanceDisplayCondition : DistanceDisplayCondition
-
Default Value:
undefined
eyeOffset : Cartesian3
x
points towards the viewer's right, y
points up, and
z
points into the screen. Eye coordinates use the same scale as world and model coordinates,
which is typically meters.
An eye offset is commonly used to arrange multiple billboards or objects at the same position, e.g., to arrange a billboard above its corresponding 3D model.
Below, the billboard is positioned at the center of the Earth but an eye offset makes it always appear on top of the Earth regardless of the viewer's or Earth's orientation.
![]() |
![]() |
b.eyeOffset = new Cartesian3(0.0, 8000000.0, 0.0);
heightReference : HeightReference
-
Default Value:
HeightReference.NONE
horizontalOrigin : HorizontalOrigin
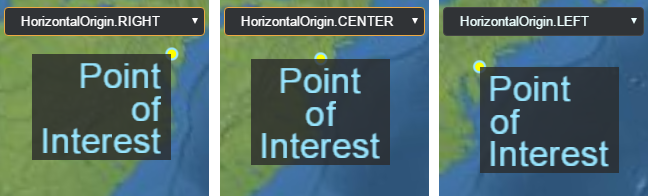
Example:
// Use a bottom, left origin
b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT;
b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
Gets or sets the image to be used for this billboard. If a texture has already been created for the given image, the existing texture is used.
This property can be set to a loaded Image, a URL which will be loaded as an Image automatically, a canvas, or another billboard's image property (from the same billboard collection).
Example:
// load an image from a URL
b.image = 'some/image/url.png';
// assuming b1 and b2 are billboards in the same billboard collection,
// use the same image for both billboards.
b2.image = b1.image;
pixelOffset : Cartesian2
x
increases from
left to right, and y
increases from top to bottom.
default ![]() |
b.pixeloffset = new Cartesian2(50, 25); ![]() |
pixelOffsetScaleByDistance : NearFarScalar
NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's pixel offset scale remains clamped to the nearest bound. If undefined,
pixelOffsetScaleByDistance will be disabled.
Examples:
// Example 1.
// Set a billboard's pixel offset scale to 0.0 when the
// camera is 1500 meters from the billboard and scale pixel offset to 10.0 pixels
// in the y direction the camera distance approaches 8.0e6 meters.
b.pixelOffset = new Cesium.Cartesian2(0.0, 1.0);
b.pixelOffsetScaleByDistance = new Cesium.NearFarScalar(1.5e2, 0.0, 8.0e6, 10.0);
// Example 2.
// disable pixel offset by distance
b.pixelOffsetScaleByDistance = undefined;
position : Cartesian3
true
, this billboard is ready to render, i.e., the image
has been downloaded and the WebGL resources are created.
-
Default Value:
false
1.0
does not change the size of the billboard; a scale greater than
1.0
enlarges the billboard; a positive scale less than 1.0
shrinks
the billboard.
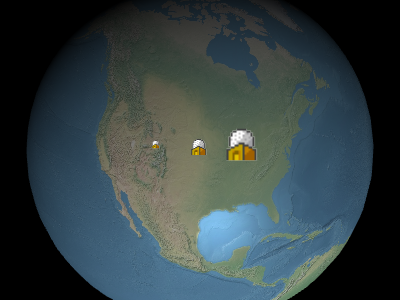
From left to right in the above image, the scales are
0.5
, 1.0
,
and 2.0
.
scaleByDistance : NearFarScalar
NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's scale remains clamped to the nearest bound. If undefined,
scaleByDistance will be disabled.
Examples:
// Example 1.
// Set a billboard's scaleByDistance to scale by 1.5 when the
// camera is 1500 meters from the billboard and disappear as
// the camera distance approaches 8.0e6 meters.
b.scaleByDistance = new Cesium.NearFarScalar(1.5e2, 1.5, 8.0e6, 0.0);
// Example 2.
// disable scaling by distance
b.scaleByDistance = undefined;
-
Default Value:
true
true
to size the billboard in meters;
otherwise, the size is in pixels.
-
Default Value:
false
translucencyByDistance : NearFarScalar
NearFarScalar#nearValue
and
NearFarScalar#farValue
while the camera distance falls within the lower and upper bounds
of the specified NearFarScalar#near
and NearFarScalar#far
.
Outside of these ranges the billboard's translucency remains clamped to the nearest bound. If undefined,
translucencyByDistance will be disabled.
Examples:
// Example 1.
// Set a billboard's translucency to 1.0 when the
// camera is 1500 meters from the billboard and disappear as
// the camera distance approaches 8.0e6 meters.
b.translucencyByDistance = new Cesium.NearFarScalar(1.5e2, 1.0, 8.0e6, 0.0);
// Example 2.
// disable translucency by distance
b.translucencyByDistance = undefined;
verticalOrigin : VerticalOrigin
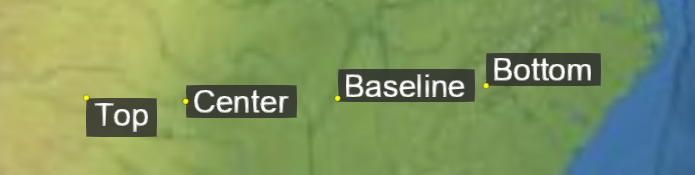
Example:
// Use a bottom, left origin
b.horizontalOrigin = Cesium.HorizontalOrigin.LEFT;
b.verticalOrigin = Cesium.VerticalOrigin.BOTTOM;
Methods
computeScreenSpacePosition(scene, result) → Cartesian2
x
increases from
left to right, and y
increases from top to bottom.
Name | Type | Description |
---|---|---|
scene |
Scene | The scene. |
result |
Cartesian2 | optional The object onto which to store the result. |
Returns:
Throws:
-
DeveloperError : Billboard must be in a collection.
Example:
console.log(b.computeScreenSpacePosition(scene).toString());
See:
Name | Type | Description |
---|---|---|
other |
Billboard | The billboard to compare for equality. |
Returns:
true
if the billboards are equal; otherwise, false
.
Sets the image to be used for this billboard. If a texture has already been created for the given id, the existing texture is used.
This function is useful for dynamically creating textures that are shared across many billboards. Only the first billboard will actually call the function and create the texture, while subsequent billboards created with the same id will simply re-use the existing texture.
To load an image from a URL, setting the Billboard#image
property is more convenient.
Name | Type | Description |
---|---|---|
id |
String | The id of the image. This can be any string that uniquely identifies the image. |
image |
HTMLImageElement | HTMLCanvasElement | String | Resource | Billboard.CreateImageCallback | The image to load. This parameter can either be a loaded Image or Canvas, a URL which will be loaded as an Image automatically, or a function which will be called to create the image if it hasn't been loaded already. |
Example:
// create a billboard image dynamically
function drawImage(id) {
// create and draw an image using a canvas
const canvas = document.createElement('canvas');
const context2D = canvas.getContext('2d');
// ... draw image
return canvas;
}
// drawImage will be called to create the texture
b.setImage('myImage', drawImage);
// subsequent billboards created in the same collection using the same id will use the existing
// texture, without the need to create the canvas or draw the image
b2.setImage('myImage', drawImage);
Name | Type | Description |
---|---|---|
id |
String | The id of the image to use. |
subRegion |
BoundingRectangle | The sub-region of the image. |
Throws:
-
RuntimeError : image with id must be in the atlas
Type Definitions
Cesium.Billboard.CreateImageCallback(id) → HTMLImageElement|HTMLCanvasElement|Promise.<(HTMLImageElement|HTMLCanvasElement)>
Name | Type | Description |
---|---|---|
id |
String | The identifier of the image to load. |